7 Essential Features of Visual Studio Code for Web Developers
Optimise your web development workflow using VS Code’s most powerful features and extensions.
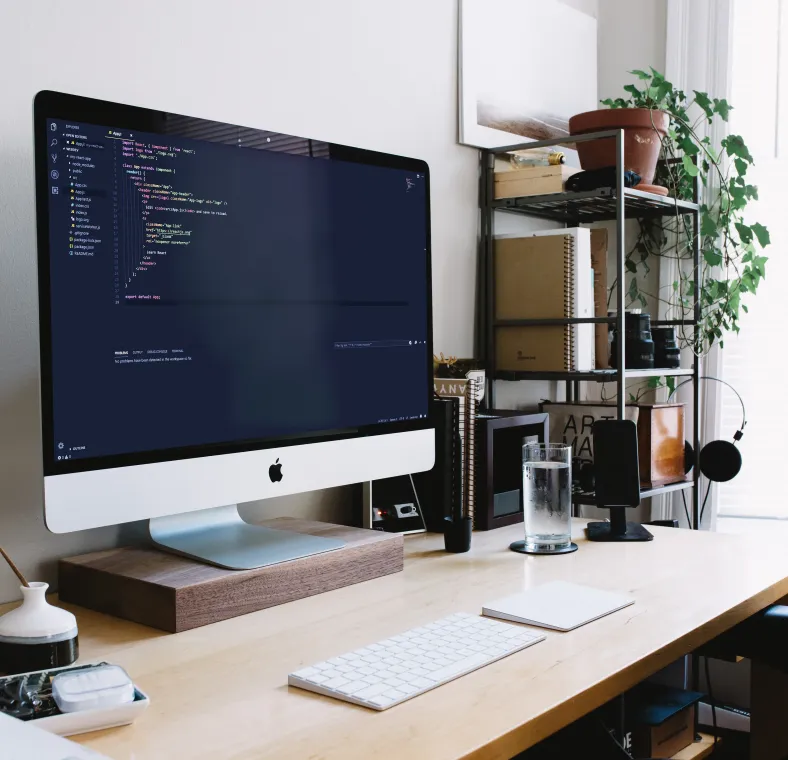
Published on
Mar 19, 2019
Read time
9 min read
Introduction
Whether you’re a professional web developer or you’re just getting started, the benefits of a faster workflow can be fantastic. In this article, we’ll dive into how to set-up an optimal workflow using Visual Studio Code.
Visual Studio Code comes with a lot of great features built-in, but add in the large (and growing) pool of extensions and you end up with thousands of ways to customise your experience.
While this is one of VS Code’s core strengths, it can also be overwhelming — especially for newer users. This article intends to cut through the noise. In it, I’ll share the most powerful techniques I use every day to work as quickly and effectively as possible.
The article is intended for two main types of people:
- Beginners, who are just starting out with VS Code and want to make sure they’re using the same tools as the pros.
- Intermediate users, who know VS Code quite well, but still feel they like they could make some improvements to their workflow.
Backstory: Why VS Code?
Since its release in 2015, Microsoft’s Visual Studio Code has quickly established itself as the most popular code editor out there. In the last two years, evidence from Google’s search trends would indicate that interest in VS Code (in red, below) has overtaken that of all other major text editors:
Worldwide data from Google Trends.
Though every editor has its unique strengths, VS Code is arguably the most popular because it’s more customisable, more regularly updated, and boasts a more diverse ecosystem of extensions than its competitors. Together, these features allow VS Code to provide an extremely fast workflow for developers — and now we’ll jump into how.
If you’ve spent time watching tutorial videos or working with other developers, you’ve likely seen several of these features in-action. The following are my go-to features for any serious web development project.
If you’re new to VS Code, you can install a copy here.
1. Emmet Abbreviations
These useful shortcuts come built-in to VS Code, and they make writing HTML (and CSS) a lot faster.
Preparing Your HTML File
If you type html
in any file, you’ll get a handful of options to fill in the initial data necessary for your file. Navigate through the options by pressing the up
or down
arrows, then press tab
to expand the shortcut, and you’ll see something like this.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body></body>
</html>
You can also find out more information about any particular option before you expand it, by pressing CNTRL + Space
or CMD + Space
.
Adding Tags
You can quickly create any HTML tags, define their ID, class names, siblings and children by knowing a few quick-to-learn abbreviations.
For example, if you want to create an unordered list with three entries, each with a unique class name, you could type ul>li.item$*3
, and you’ll end up with:
<ul>
<li class="item1"></li>
<li class="item2"></li>
<li class="item3"></li>
</ul>
In this example, we used the following shorthand symbols:
>
specifies that the next tag should be a child of the previous one..
specifies that the text that follows is a class name.$
is used to print a unique number (counting up from1
).- Finally,
*
allows you to quickly multiply a tag, to produce as many as you want.
You can get a full list of Emmet shortcuts using the official documentation, while the easiest place to get started is probably this cheat sheet.
Adding Dummy Text
One of the most useful Emmet abbreviations for web development allows you to insert a chosen amount of dummy text. To insert 100 words, simply write lorem100
and press tab
. For 1,000 words, write lorem1000 + tab
— and so on.
Emmet for JSX
If you use React’s JSX or another alternative to HTML, you can still use Emmet abbreviations. You just have to enable them manually.
To do that, press CTRL + ,
to open your settings and click the curly braces symbol {}
in the top right corner to open up settings.json
. When that’s open, simply add the following code:
{
"emmet.syntaxProfiles": {
"javascript": "jsx",
"xml": {
"attr_quotes": "single"
}
}
}
2. The Integrated CLI (Command Line Interface)
To save switching between windows, VS Code offers an integrated terminal or CLI. Simply, press CNTRL + '
or CMD + '
to open it up, and the same command to close it. It will automatically open in the directory you have open in VS Code, which saves the navigation step required for operations in a standard terminal.
This makes it easy to install NPM or Yarn dependencies, commit files to Git, and push files to Github — as well as anything else you might want to do via the command line!
A Note For Windows Users
If you’re using Windows, I strongly recommend installing Git for Windows. This will allow you to a number of commands familiar to Mac and Linux users, as well as allowing you to run Git from the command line.
Once installed, you can change your default terminal from Powershell to Git Bash by adding the following code to settings.json
:
{ "terminal.integrated.shell.windows": "C:\\Program Files\\Git\\bin\\bash.exe" }
3. ESLint
ESLint is a powerful and popular linting tool, which helps you spot errors in your code and fix them as you write and which helps you follow common best-practices. It’s also a great learning tool, since researching ESLint errors has taught me many best practices I didn’t know before, and it’s helped me understand why they are best practices.
You can add it as a node package on a per-project basis or you can use the ESLint extension within VS Code. The second option is quick and easy, and it won’t stop you using unique ESLint configurations for particular projects later on. Simply find the ESLint plugin (the one by Dirk Baeumer) and install it.
To get the most out of the extension, you’ll likely want a global version of the ESLint library installed on your computer. To do that, ensure you have Node installed. Then open your terminal (if you’re inside VS Code, press CNTRL + '
or CMD + '
) and type in the following code:
npm i -g eslint
With the extension and global library installed, you don’t need to run npm i eslint -s
on an individual project if you don't want to. Instead, just add an eslintrc
file to the main directory of your project.
To do this, you’ll need to initialise NPM. Make sure you’re in your project’s main directory in the terminal and type npm init
. You can then create your eslintrc
file by typing eslint --init
and following through the steps. (If you’re not sure which style-guide to use, I recommend AirBnB’s.)
Once you’re happy with your ESLint settings, you can make sure that any automatic fixes are applied on saving, by adding the following to settings.json
:
{ "eslint.autoFixOnSave": true }
Another Note for Windows Users: Pesky Linebreaks
By default, line-breaks on Mac and Linux use a simple line feed (LF): \n
. Windows, however, uses a carriage return line feed (CRLF): \r\n
.
There are two quick solutions to this. First, you could change the default linebreak settings from unix
to windows
. To do this, go to your eslintrc
file and add "linebreak-style": "windows"
under the rules
object.
Or if you’re like me, and you think \r\n
seems unnecessary when \n
will suffice (or you’re collaborating with Mac or Linux users), you can change the default linebreak to LF, by adding the following code to settings.json
:
{ "files.eol": "\n" }
4. Prettier
Prettier is an opinionated code-formatter. It prescribes a certain formatting style as the correct one, but its popularity is making its rules something of an established standard for JavaScript, CSS, and increasing numbers of other languages.
There is overlap between Prettier and ESLint, but ultimately ESLint is more focused on spotting errors and Prettier is more focused on correcting formatting. They work great together, and there’s an integration to help make this as smooth as possible.
The extension we want is “Prettier — Code formatter” by Esben Peterson. To enable the ESLint integration, add the following code to settings.json
:
{ "prettier.eslintIntegration": true }
Format on Save
Once it’s installed, we can press Shift + Alt + F
or Shift + Option + F
and Prettier will immediately shift our JavaScript (or CSS) into place.
I prefer to format automatically every time I save. To make that the default, add this code to settings.json
:
{ "editor.formatOnSave": true }
Other Languages
And you can also use Prettier for more languages than just JavaScript or CSS. It has a growing list of community plugins, which support languages including Java, Ruby, and — WordPress developers rejoice — PHP. (These don’t come out-of-the-box, so you’ll have to install them separately.)
5. Multi-Cursor Shortcuts
The ability to edit with multiple cursors can be a huge time saver. To use this most effectively requires memorisation of a handful of commands, but they’ll soon become second nature.
The Basics
To manually add new cursors, hold Alt
or Option
and then click wherever you want a new cursor. You can go back to a single cursor by pressing Escape
. With those two commands, you can save a lot of time. But there’s more!
Column Selection
By holding down Shift + Alt
or Shift + Option
while you drag your mouse, a new cursor will be added to the end of each selected line.
Immediately Above or Below
To add new cursors immediately above or below the current cursor, you can use CTRL + Alt
or CMD + Option
and then add the up
or down
arrow keys.
Select All Identical Code
By highlighting a section of code and pressing CTRL + Shift + L
or CMD + Shift + L
, you can immediately add a new cursor at the end of every identical section of code.
Select Identical Code One-by-One
In cases where you don’t want all identical code, you can use CTRL + D
or CMD + D
to highlight identical text one-by-one.
Note: Keymap Extensions
If you’ve got accustomed to another text editor’s set of keybindings, chances are there’s an extension for that. Instead of losing time learning a new set of keybindings, go to Preferences > Keymaps to see a list of the relevant extensions.
Note that many of the shortcuts described in this article will change when you install a different keymap. If you ever want to go back to the default, simply find the relevant extension(s) and disable them.
6. Text Wrap
There aren’t many cases where I’d prefer my text to run off the screen, forcing me to use the dreaded horizontal scroll. To toggle wrapping on or off on a per-file basis, simply press ALT + Z
.
Or, if you’re like me and almost never want to turn text-wrapping off, you can make it the default. Inside settings.json
, simply paste in the following code, and your text will wrap by default:
{ "editor.wordWrap": "on" }
7. Execute and Debug JavaScript
There are several great options for executing and debugging JavaScript within Visual Code studio.
Quokka.js
Quokka is a freemium tool that helps you see what’s going on in your JavaScript in real time. It describes itself as a “live scratchpad”, and it gives you a quick visual aid to check that your code is doing what it’s supposed to.
Once it’s been installed, press CNTRL + K, Q
or CMD + K, Q
to start (or restart) Quokka on an existing JavaScript file.
Debugger for Chrome
The best choice for debugging JavaScript within VS Code is Microsoft’s own “Debugger for Chrome” extension. How it works can be a little complex, so I recommend you take a look at the official documentation if you want to learn more. Suffice to say that this is a powerful tool, and it was a real eye-opener for me when I discovered it — it will take you a lot further than console.log()
!
Code Runner
If the above options seem a little involved and you’d prefer a simple way to run code snippets or files, I recommend the Code Runner extension by Jun Han. It covers a large number of languages, and with a simple command ( CTRL + Alt + N
or CMD + Option + N
) you can get see the output of your code at the bottom of the screen. For JavaScript, it’s like having a browser console integrated into VS Code.
Conclusion
The features discussed in this article are extremely useful for any web development set-up, and I hope they’ve helped you make your VS Code workflow as fast as possible — or, if you’re new to VS Code, get a strong set-up from the start.
Other Resources
It is a credit to VS Code’s developers that it has so much to offer, and there are countless other ways to augment and customise your experience even further.
If you’d like to find out more cool features, I recommend reading:
settings.json
Lastly, if you’ve followed this article and want to check that your settings.json
file looks the same as mine, you should end up with something like this.
{
"emmet.syntaxProfiles": {
"javascript": "jsx",
"xml": {
"attr_quotes": "single"
}
},
"terminal.integrated.shell.windows": "C:\\Program Files\\Git\\bin\\bash.exe",
"eslint.autoFixOnSave": true,
"files.eol": "\n",
"prettier.eslintIntegration": true,
"editor.formatOnSave": true,
"editor.wordWrap": "on",
"workbench.colorTheme": "Ayu One Dark Pro | Mirage"
}
Related articles
You might also enjoy...
Switching to a New Data Structure with Zero Downtime
A Practical Guide to Schema Migrations using TypeScript and MongoDB
7 min read
How to Automate Merge Requests with Node.js and Jira
A quick guide to speed up your MR or PR workflow with a simple Node.js script
7 min read
Automate Your Release Notes with AI
How to save time every week using GitLab, OpenAI, and Node.js
11 min read