How to Add Bugsnag to Strapi v4
Learn how to integrate Bugsnag into a Strapi v4 project via a global middleware function
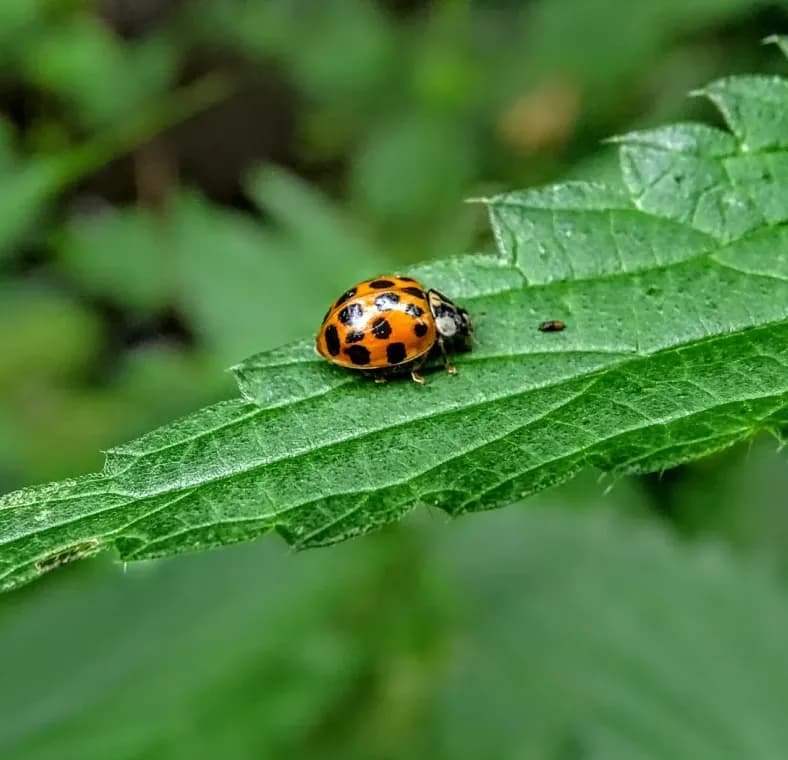
Published on
Jul 30, 2022
Read time
2 min read
Introduction
In this tutorial, we’ll explore how to add Bugsnag to a Strapi project via a global middleware function.
Bugsnag is a useful tool for monitoring and reporting bugs.
Strapi is a powerful headless CMS. Its most recent version, v4, introduced significant changes to the structure of the project, meaning that older tutorials (such as this one, for v3) are no longer relevant.
Creating a new Strapi Project
To create a new Strapi project, run:
npx create-strapi-app@latest my-project
Installing Dependencies
Navigate into your new project, and install the following dependencies. Strapi is built using Koa, so we’ll need to use Bugsnag’s Koa plugin.
npm install --save @bugsnag/js @bugsnag/plugin-koa
Adding Bugsnag via a new Middleware
In src/index.js
, you’ll see an empty bootstrap
function. In here, we can initiate Bugsnag.
async bootstrap({ strapi }) {
Bugsnag.start({
apiKey: process.env.BUGSNAG_KEY,
plugins: [BugsnagPluginKoa],
});
}
Make sure to install your key somewhere safe. In this example, we’ve added a environment variable called BUGSNAG_KEY
to our .env
file. This can be generated inside the Bugsnag UI (make sure to select the Koa template).
Next, we’ll add a new script to our package.json
, which will help us use Strapi’s generate
helper.
"scripts": {
"generate": "strapi generate",
// other scripts
}
Now we can run npm run generate
. Choose middleware
, call it “bugsnag”, and select Add middleware to root of project
. You’ll now see a new file has been created atsrc/middlewares/bugsnag.js
, which will look something like this:
"use strict";
module.exports = (config, { strapi }) => {
return async (ctx, next) => {
strapi.log.info("In bugsnag middleware.");
await next();
};
};
In here, we can use Bugsnag to handle requests and errors. We’ll call strapi server use
and then handle the errors inside the catch
of a try/catch
block.
"use strict";
const Bugsnag = require("@bugsnag/js");
module.exports = (_config, { strapi }) => {
strapi.server.use(async (ctx, next) => {
const middleware = Bugsnag.getPlugin("koa");
try {
await middleware.requestHandler(ctx, next);
} catch (error) {
middleware.errorHandler(error, ctx);
throw error;
}
});
};
Finally, we need to tell Strapi when to trigger this new middleware. This can be done inside config/middlewares.js
. Our new middleware is called "global::bugsnag"
and it should be activated as early as possible. In our new project, it can go right at the top of the array:
module.exports = ({ env }) => [
"global::bugsnag",
"strapi::errors",
// other middlewares
];
You should now have a working Bugsnag integration!
Testing Your Integration
To test your integration, you can call Bugsnag.notify(new Error("test"))
in the codebase and you should see this error logged in your Bugsnag dashboard. This could be done, for example, inside another custom middleware.
Related articles
You might also enjoy...
Switching to a New Data Structure with Zero Downtime
A Practical Guide to Schema Migrations using TypeScript and MongoDB
7 min read
How to Automate Merge Requests with Node.js and Jira
A quick guide to speed up your MR or PR workflow with a simple Node.js script
7 min read
Automate Your Release Notes with AI
How to save time every week using GitLab, OpenAI, and Node.js
11 min read