Backend Development: an Introduction for Frontend Developers
First Steps in Node.js and Express.js
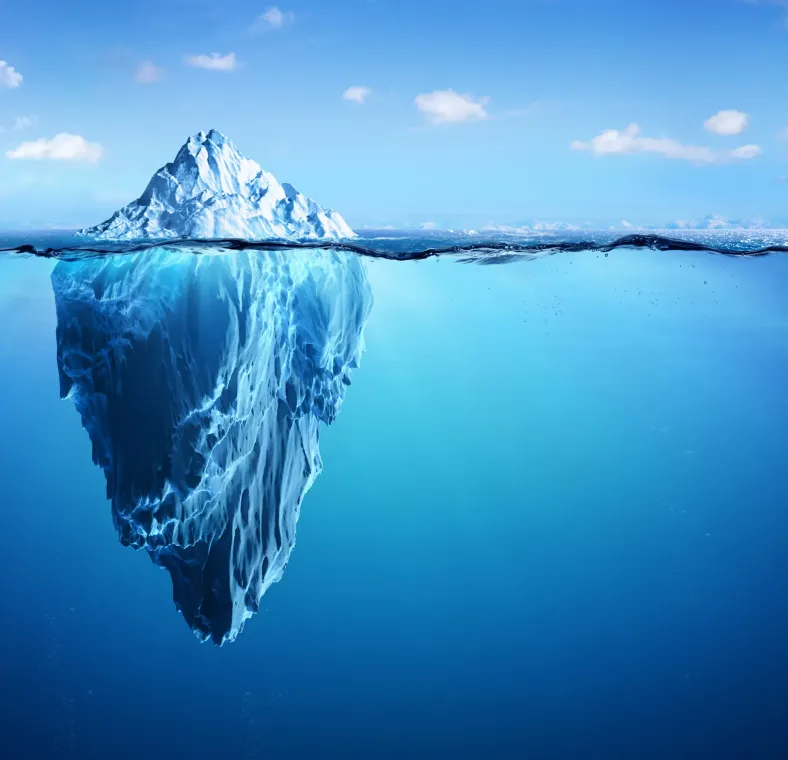
Published on
May 29, 2019
Read time
7 min read
Introduction
A few months ago, I found myself googling ‘is backend development harder than frontend development?’ I had reached a point with front-end development where I was able to solve most problems I encountered — with a bit of research. But, as a self-taught developer, backend programming had remained a mystery!
I was relieved to discover that the problem went both ways: for every frontend developer intimidated by Node.js, Python or Ruby, there’s a backend developer daunted by CSS, Vue or React. Often, it just depends on what you learnt first.
In this article, we’ll explore how to set up your very first backend server. But first, what is it about frontend and backend development that suits some people and terrifies others?
The Frontend
The frontend has the advantage of immediate visual feedback. But it also has a host of related considerations — such as UI and UX design — that might send some backend developers running.
It’s easy to get started with HTML and CSS, but much harder to master the full range of technologies that have become standard in modern frontend programming. Throw in added complexities like cross-browser compatibility, and you can see why some people would rather stick to server-side code.
The Backend
As for the backend, it can be a greater challenge to get started with because it’s more difficult to see the results of your code. It tends to depend more on using the command line, and it’s also more dependent on jargon: anyone can work out what a paragraph tag does, but GET and POST requests— although integral to backend development and the web — are not quite so intuitive to understand.
On the flipside, you don’t have to worry about end-users in the same way and, once you feel more comfortable with backend, you realise that a lot of core tasks — for simpler projects, at least — are the same from one application to the next: creating a server, setting up routing, connecting to and managing a database, and so on.
Who’s This Article For?
I’m writing this article for anyone in a similar position to me, who first became comfortable with frontend development and now wants to add backend development to their repertoire.
We’ll be focusing on Node.js and Express.js: the E and the N in the popular MEAN and MERN tech-stacks. But the concepts discussed should be useful for anyone with some frontend knowledge, whether they’re looking to get their head around backend development in JavaScript, Ruby or Python.
Backend Jargon
In the next section, we’ll use Node.js to set up a really simple server. But before digging into the code, it’s useful to understand a handful of other concepts.
For me, the key difference between picking up frontend and backend skills for the first time was that backend required far more jargon in order to get started. Though you’ve no doubt encountered terms like server, HTTP, GET and POST — using these concepts in backend code requires more than a superficial understanding. So, here’s a speedy tour of some of the terms you’ll need to know.
What is a Server?
A server is a computer program or device that provides functionality for other programs or devices, known as “clients”. In web development, that server uses HTTP to serve files to users which render web pages. This happens in response to requests which are forwarded from the HTTP clients of users’ computers: when you type in a URL to your browser’s address bar, you are sending an HTTP request.
What is HTTP?
HTTP stands for ‘Hypertext Transfer Protocol’, and it is the system of rules that underlies communication between clients and servers on the web. You may have encountered two HTTP methods, GET and POST requests, in frontend code when programming forms, but their use in backend code is much wider. Node.js also has an in-built HTTP module, which we’ll be using shortly to create our server.
Requests and Responses
The two most common arguments for Node’s http.createServer()
method and many other backend methods are known as req
and res
, which stand for ‘request’ and ‘response’). These are objects with properties and methods that we can edit in order to customise our HTTP requests.
In simple terms, req
is what we send out and res
is what we get back:
req
is the object which contains information about the client HTTP request that raised the eventres
is used to send back the desired server HTTP response.
In Express.js, the functions that grant access to req
and res
are known as middleware functions, and you can read more about those here.
HTTP Methods
There are also a handful of HTTP methods, and it will be useful to understand the two most common types:
- GET requests are used to request data from a specified resource. They can be bookmarked and cached, and they remain in a browser’s history. If used to submit a form, all the form data is visible in the URL — that’s why they should never be used for sensitive data.
- POST requests are used to send data to a server, allowing resources to be created or updated. They cannot be bookmarked or cached, and they will not appear in a browser’s history.
HTTP Response Status Codes
For a simple server, we also need to know a little about HTTP status codes. These are messages used to indicate whether a specific HTTP request has been successfully completed.
Even most non-developers have encountered 404 “Not Found”, which appears when you try to access a URL that doesn’t exist. And if you’ve ever been involved with SEO, you might be aware of codes like 308 “Permanent Redirect”, when a page has been permanently moved to another URL.
For our server, we want to use 200 “OK”, indicating that an HTTP request has succeeded.
What is a Hostname and a Port?
Finally, we’ll also be choosing a hostname and port, so it’s also useful to understand a bit more about these.
A hostname is a label used to identify a device in a network, allowing computers to communicate with one another. A hostname can be translated into an IP address, which allows the computer to be uniquely identified on the internet.
In this example, we’ll be using the special 127.0.0.1 IP address, also known as the localhost. The localhost specifically describes the host computer that accesses it: every computer uses this address as their own, and unlike regular IP addresses, it doesn’t let the computer communicate with other devices. It is considered a “loopback” address because the information sent to it is routed back to the local machine.
A port is an endpoint of communication. It is a number from 0 to 65535 (with the range 0 to 1024 reserved for system ports). You might often use http://localhost:3000
to access a development server for web applications, and the number 3000
represents the port. Ports can be used to “listen” for service requests from clients: in our example, the port will listen for connections to the server.
Setting Up Your First Server
To set up your first Node.js server, first, ensure you’ve got it installed on your machine. Create a new directory for your project and, inside that, an empty JavaScript file called index.js
. Finally, navigate to that directory in your terminal and run npm init
.
You can accept all the defaults, though make sure that the entry point is index.js
. This will create a package.json
file in the root directory of your project. We’re now ready to start writing JavaScript!
Using Node.js
First, we’ll need to require in the HTTP module using const http = require('http')
. We can then set the hostname to '127.0.0.1'
, choose a port and create our server using the http.createServer()
method.
Within this function, we’ll set the statusCode
property of the result to 200
(meaning “OK”). We’ll also tell the server that our result is plain text, using the setHeader
method, and we’ll provide our desired text via the end
method.
Finally, we’ll set our server to listen to our chosen port and hostname, and we’ll log a message to the console so that we know everything’s working correctly.
const http = require("http");
const hostname = "127.0.0.1";
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader("Content-Type", "text/plain");
res.end("Hello World\n");
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
To activate your server, navigate to your project directory in the terminal and type node .
. You can then open up [http://127.0.0.1:3000](http://127.0.0.1:3000)
in a browser and you should see the words ‘Hello World’. Success!
Using Express.js
The example above shows you how to set up a simple server using only the in-built HTTP module of Node.js, but it is more common to use Express.js for core backend tasks.
Express.js offers a number of advantages over Node.js by itself, including static file serving, view caching, easy integration with a number of popular templating engines (such as Pug) and numerous useful routing features. The boilerplate code required to set up a simple server is also a little more concise than the HTTP alternative.
To get started with Express, navigate to your project directory in the terminal: type npm init
to initialise your project with a package.json
file, then type npm i express
to install express. You can copy and paste the following code into index.js and you’ll have a running server:
const express = require("express");
const app = express();
const hostname = "127.0.0.1";
const port = 3000;
app.get("/", (req, res) => res.send("Hello World!"));
app.listen(port, hostname, () =>
console.log(`Server running at http://${hostname}:${port}/`)
);
The hostname
, port
and listen
method are identical to the previous example, but the get
method is a feature of Express. One of the advantages of Express over plain Node.js is that Express has separate handlers HTTP methods such as get
, post
, put
and so on. It provides a simple way to route HTTP GET requests to a specified path: in this case, the root.
Nodemon
Lastly, for server-side code, it’s very common to use the nodemon module (pronounced node-mon), which automatically restarts your server any time the source code changes. To install it, type npm i -g nodemon
and then, instead of using node index
to run your server, you can use nodemon index
.
Next Steps
I hope this article is useful for anyone looking to write server-side code for the first time, and — in particular — that it clarified some of the jargon that can prevent people from jumping into backend development.
If you’re interested in learning more, I recommend following the official ‘Getting Started’ section of the Express.js site. Or, for an extremely useful and comprehensive introduction to Node.js and Express, take a look at MDN’s article on the topic.
If you’d like to find out more about HTML templating, I cover Pug (commonly used with Express) and a handful of other options in another article. And if you’re interested in learning about other ways to use Node.js, check out my tutorial on creating a web scraper. If you have any questions or feedback, please leave a comment!
Related articles
You might also enjoy...
Switching to a New Data Structure with Zero Downtime
A Practical Guide to Schema Migrations using TypeScript and MongoDB
7 min read
How to Automate Merge Requests with Node.js and Jira
A quick guide to speed up your MR or PR workflow with a simple Node.js script
7 min read
Automate Your Release Notes with AI
How to save time every week using GitLab, OpenAI, and Node.js
11 min read